Blog > Python Programming > How to Stand Out in a Python Coding Interview?
How to Stand Out in a Python Coding Interview?
Introduction
You’ve moved past the initial phone screen and now face the challenge of demonstrating your coding skills through various formats like HackerRank exercises, take-home assignments, or onsite whiteboard interviews. This is your opportunity to showcase not only your problem-solving abilities but also your proficiency in writing clean, production-level code.
Interviews require a deep understanding of Python’s built-in functionalities and libraries. Demonstrating this knowledge shows potential employers that you can work efficiently and avoid reinventing the wheel. At Heicoders Academy, we’ve identified key tools that impress us in coding interviews. This article will guide you through Python’s built-ins, native data structure support, and the robust standard library.
Learning Objectives
In this article, you’ll learn how to:
- Use enumerate() to iterate over both indices and values.
- Debug code effectively with breakpoint().
- Format strings efficiently using f-strings.
- Sort lists with custom arguments.
- Utilize generators to conserve memory instead of list comprehensions.
- Define default values for dictionary key lookups.
- Leverage the standard library to generate lists of permutations and combinations.
Iterate With enumerate() Instead of range()
In coding interviews, you might need to iterate over a list and access both indices and values. For example, the FizzBuzz problem:
Replace integers divisible by 3 with “fizz”, by 5 with “buzz”, and by both with “fizzbuzz”. Using range():
A more elegant solution uses enumerate():
With enumerate(), you get a counter and the element value, starting from 0 by default. You can also set a custom start value:
Output:
Using enumerate() makes your code more readable and concise, especially when you need both index and value.
Use List Comprehensions Instead of map() and filter()
Python’s creator, Guido van Rossum, suggested moving away from map() and filter() in favor of list comprehensions due to their readability and simplicity.
Let’s compare map() with a list comprehension:
Both methods yield the same result, but list comprehensions are generally easier to read.
Similarly, let’s look at filter() versus list comprehensions:
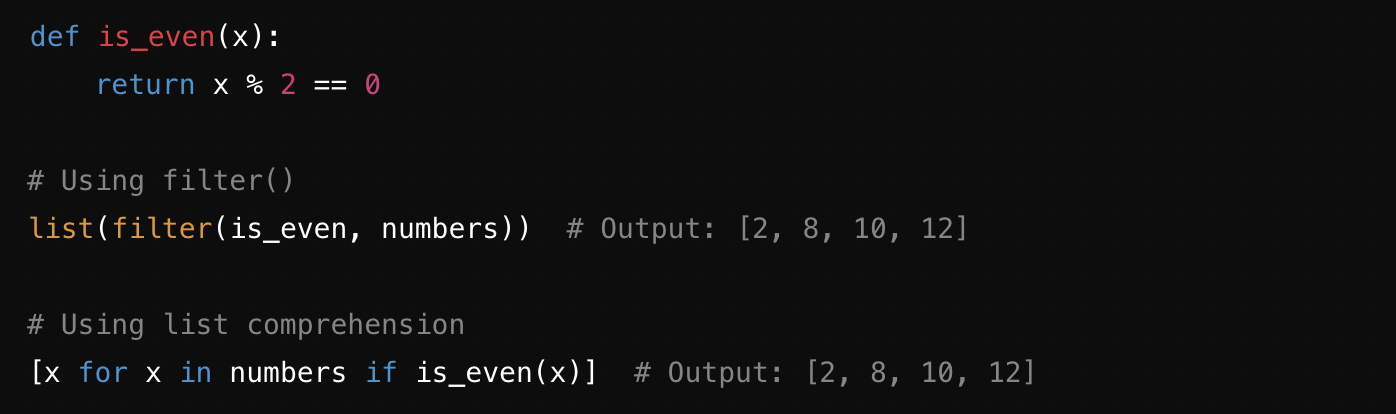
Again, both approaches produce the same result, but list comprehensions are more intuitive, especially for beginners. Using list comprehensions in coding interviews demonstrates familiarity with common Python practices and enhances code readability.
Debug With breakpoint() Instead of print()
Using print() to debug can be helpful for small problems but quickly becomes cumbersome, especially in a coding interview. Instead, use a debugger to save time and demonstrate your ability to use professional tools.
For Python 3.7 and later, you can simply call breakpoint():
This will invoke pdb, the default Python debugger. For Python 3.6 and older, you can achieve the same by explicitly importing pdb:
Using a debugger like pdb makes it easier to inspect and control the flow of your program, showcasing your proficiency in efficient debugging techniques.
Format Strings With f-Strings
Python offers various ways to handle string formatting, but for Python 3.6+, f-strings are the recommended approach, especially in coding interviews. F-strings support string formatting mini-language and powerful string interpolation, allowing you to include variables and expressions evaluated at runtime.
Example:
F-strings provide a concise way to include variables and format strings in one operation. However, be cautious when outputting user-generated values, as it may introduce security risks. In such cases, consider using Template Strings.
Sort Complex Lists With sorted()
Sorting is a common task in coding interviews, and using sorted() is often the best approach unless you’re specifically asked to implement a sorting algorithm.
For simple sorting, sorted() can handle lists of numbers or strings:
By default, sorted() sorts in ascending order. Use the reverse argument for descending order.
For more complex sorting, use the key argument to define custom sorting rules. For example, sorting a list of dictionaries by a specific key:
By using a lambda function that returns the desired sort key, you can sort complex data structures easily and efficiently.
Save Memory With Generators
List comprehensions are convenient but can lead to high memory usage.
For instance, finding the sum of the first 1000 perfect squares with a list comprehension:
This approach creates a list of all perfect squares, which is inefficient for large ranges, causing memory issues.
Instead, use a generator expression to save memory:
Using parentheses converts the list comprehension into a generator expression. Generators compute values on demand, holding only one element in memory at a time. This allows efficient handling of large sequences.
For example, summing the first 1,000,000 perfect squares:
This method prevents memory overflow by generating each value only when needed, unlike list comprehensions that store all values at once. Generators are ideal for processing large data sequences efficiently.
Define Default Values in Dictionaries With .get() and .setdefault()
When working with dictionaries, you often need to add, modify, or retrieve items that may not exist. Python offers elegant methods to handle these tasks efficiently.
Imagine you have a dictionary representing a student’s profile, and you want to retrieve the student’s grade. One approach is to explicitly check for the key with a conditional:
This can be simplified using .get():
Using .get() handles the key existence check and returns a default value if the key is missing.
To update the dictionary with a default value while retrieving it, use .setdefault():
.setdefault() checks if the key exists and returns its value; otherwise, it sets the key to the default value and returns it. This method makes the code more concise and readable.
Generate Permutations and Combinations With itertools
Interviewers often use real-life scenarios to make coding interviews more relatable. Imagine you’re at an amusement park and need to find every possible pair of friends that could sit together on a roller coaster. Generating these pairs can be tedious with nested loops, but the itertools library simplifies this task.
Permutations
itertools.permutations() creates a list of all possible orderings of input values for a given length. For example, generating all possible pairs of friends:
In permutations, the order matters, so (‘Alice’, ‘Bob’) is different from (‘Bob’, ‘Alice’).
Combinations
itertools.combinations() generates all possible groups where order does not matter. For example, generating all possible pairs of friends:
In combinations, (‘Alice’, ‘Bob’) is the same as (‘Bob’, ‘Alice’), so only one of these is included.
Practical Use
Using itertools saves time and reduces complexity when dealing with combinatorial problems in coding interviews. By leveraging these functions, you can focus on solving the core problem rather than getting bogged down in the details of generating permutations and combinations manually.
More Examples
Permutations:
Combinations:
By utilizing these tools from itertools, you can efficiently handle large datasets and complex combinations, making your code both cleaner and faster.
Conclusion: Mastering Python for Coding Interviews
You can now confidently use some of Python’s more powerful and lesser-known features in your next coding interview. While there’s always more to learn, this article has provided a solid foundation to enhance your understanding and interview performance.
In this article, you’ve learned how to leverage:
- Powerful built-in functions
- Data structures designed for common scenarios
- Feature-rich standard library packages
While coding interviews may not perfectly mimic real software development, mastering these tools will help you succeed in any programming environment. Deepening your understanding of Python will benefit your day-to-day development.
By incorporating these techniques, you’ll be better prepared to tackle interview challenges and demonstrate your proficiency in Python effectively.
If you do not have any prior experience in Python programming, and are interested to pick it up through a structured background, do check out Heicoders Academy’s AI100: Python Programming & Data Visualisation. This course are meant for beginners without any background, and is meant to equip you with all the key fundamentals in Python programming.
Those interested to go further can also consider AI200: Applied Machine Learning and AI300: Deploying Machine Learning Systems to the Cloud.
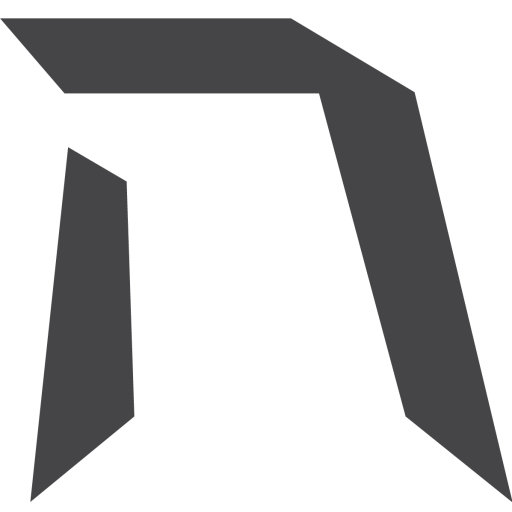
Upskill Today With Heicoders Academy
Secure your spot in our next cohort! Limited seats available.